API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/gpt-image-1-edit"
# Request payload
data = {
"prompt": "Make a picture of a 3D action figure toy, named 'Teena'. Display it in transparent blister packaging with 'Teena' in white text at the top. Action figure wears a trendy, chic outfit featuring a stylish crop top with high-waisted skinny jeans and fashionable heels. Include accessories: a smartphone with selfie stick, a small ring light, and a designer handbag beside the figure. Use minimalist cardboard packaging design in cute toy store style. Cartoonish, cute yet neat appearance.",
"image_urls": [
"https://segmind-resources.s3.amazonaws.com/output/cf5d6d3d-9be2-4538-b6b2-3d8ff11594b9-Beach-walk.png"
],
"size": "auto",
"quality": "auto",
"background": "opaque",
"output_compression": 100,
"output_format": "png",
"moderation": "auto"
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Text prompt used to generate the image.
A list of images.
Input Image.
Select image resolution. Square is the fastest to generate.
Allowed values:
Controls the visual quality of the output image.
Allowed values:
Select whether the image background should be transparent or opaque.
Allowed values:
Select the compression level for the output image (1-100).
Select the output format of the image.
Allowed values:
Controls the moderation strictness - use low for less restrictions.
Allowed values:
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
GPT-Image-1 Edit: Precision Image Editing with the Power of Language and Vision
GPT-Image-1 Edit is OpenAI’s powerful inpainting and multi-reference image editing model that brings Photoshop-like control into the realm of natural language. Built for developers, creators, and AI toolmakers, it enables detailed visual edits, image composition, and object-level replacements—all guided by intuitive prompts. Whether you're modifying product shots, refining creative assets, or combining multiple visual inputs into a single composition, GPT-Image-1 Edit makes it seamless.
How It Works
Unlike the standard image generation model, GPT-Image-1 Edit focuses on transforming existing images. It supports four core editing capabilities:
-
Inpainting with Masks: Upload an image and a corresponding mask (with an alpha channel). The model uses the prompt to fill the transparent areas of the mask with entirely new content—ideal for object replacement, background changes, or localized edits.
-
Multi-Image Composition: Upload multiple reference images and describe how they should be combined. GPT-Image-1 Edit intelligently merges them into a single, coherent image. For example, uploading a soap bar, bath bomb, and lotion can generate a photorealistic gift basket.
-
Style-Guided Transformation: Use one or more images to define the visual style, and let the model adapt another image accordingly.
-
Partial Overwrites: Provide an image and ask the model to modify specific elements—like changing the sky in a landscape or replacing a product label.
The API uses standard multipart/form-data
for image uploads and supports images up to 25MB with alpha channels for masks. Like the generation model, output can be customized in terms of format (PNG, JPEG, WebP), background transparency, and compression levels.
Use Cases and Applications
- Marketing & Product Imagery: Instantly update packaging, create seasonal variants, or adapt visuals for different campaigns
- eCommerce: Showcase products in different scenes, colors, or setups without redoing photoshoots
- Editorial: Retouch or recompose photos with better framing, lighting, or subject arrangement
- UI/UX & Game Design: Tweak icons, characters, or assets in existing compositions without starting from scratch
- Creative Tools: Build powerful AI-powered editors or creative apps with intuitive prompt-based editing
Why It Matters
GPT-Image-1 Edit blurs the line between generative and traditional design tools. It empowers creators to edit with words, reduce manual retouching time, and generate asset variants rapidly. With its fine control over visual composition and the ability to combine references, it becomes a core building block for next-gen design tools, AI-assisted workflows, and content platforms looking to scale visual operations.
Other Popular Models
sadtalker
Audio-based Lip Synchronization for Talking Head Video
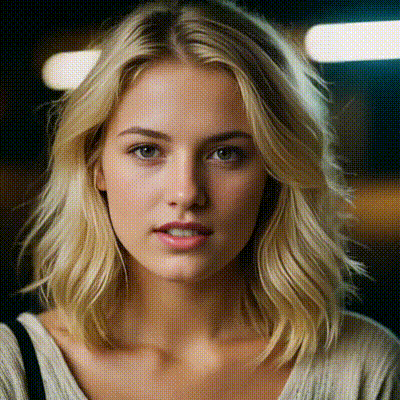
fooocus
Fooocus enables high-quality image generation effortlessly, combining the best of Stable Diffusion and Midjourney.
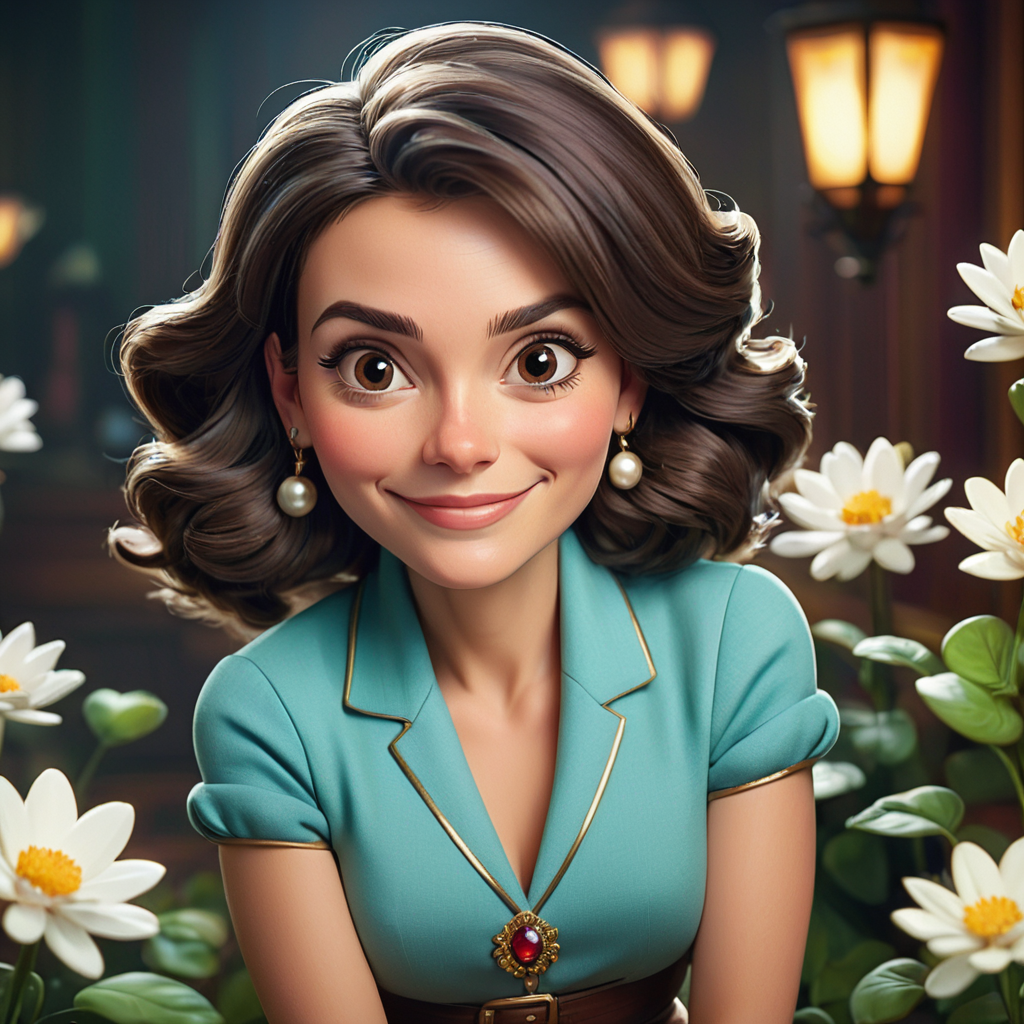
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
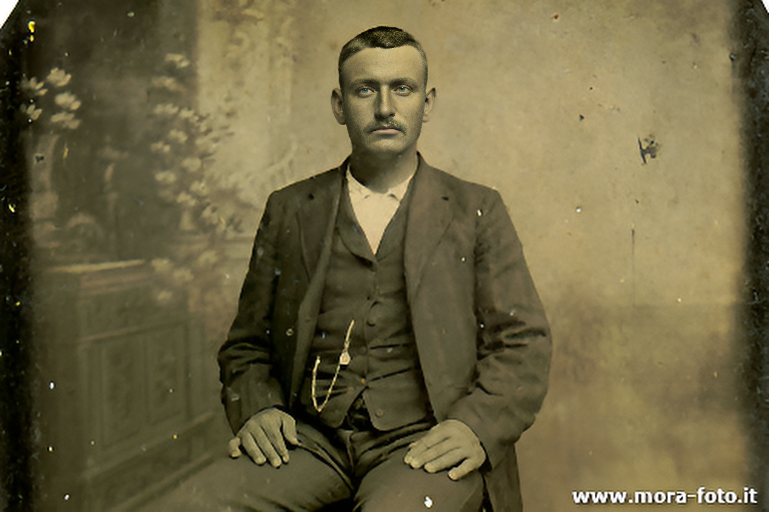
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
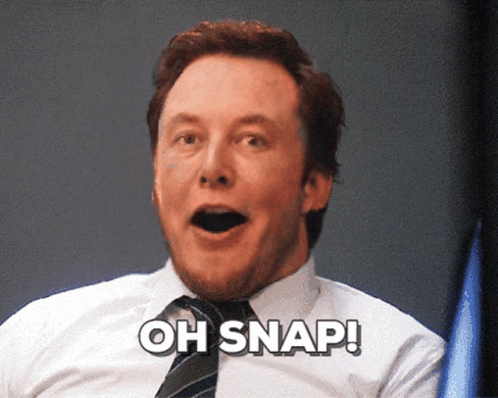