API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/ideogram-3"
# Request payload
data = {
"prompt": "A cinematic photograph of a dark forest scene viewed from a distance. In the center, a small patch of glowing plants and flowers is illuminated by a faint, focused glow, casting a soft reflection in a still stream below. The delicate neon-style white text 'Segmind' is softly lit, creating a striking contrast against the deep shadows and mist. The surrounding dense foliage and mist create a mysterious and moody atmosphere, with strong contrast between light and shadow, enhancing the emotional and atmospheric tone.",
"color_palette": {
# Either provide a named palette
"name": "MELON", # required, mutually exclusive with "members"
# Or provide custom colors
"members": [ # required, mutually exclusive with "name"
{
"color_hex": "", # required if using "members"
"color_weight": 0 # optional
}
]
},
"resolution": "1024x1024",
"rendering_speed": "DEFAULT",
"magic_prompt": "AUTO",
"negative_prompt": "No people, no animals.",
"style_codes": [],
"style_type": "GENERAL"
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
The prompt guides image creation; use detailed descriptions for specific results. Example: 'A futuristic cityscape at sunset.'
The seed ensures reproducibility; set it for consistent output, omit for variation.
Resolution affects image detail; higher values for precise detail, lower for speed.
Allowed values:
Aspect ratio formats your image; select '1x1' for square, '16x9' for widescreen.
Allowed values:
Rendering speed affects time taken; 'TURBO' for faster, 'QUALITY' for detailed results.
Allowed values:
Magic Prompt helps enhance descriptions; use 'ON' for complex scenes, 'OFF' for simplicity.
Allowed values:
Exclude elements by specifying negatives; e.g., 'No people, cars'.
Style codes customize look; use predefined codes for specific styles.
Style type influences art style; choose 'REALISTIC' for lifelike, 'DESIGN' for artistic.
Allowed values:
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Discovering Ideogram 3.0: Empowering Developers and Creators
Released on March 26, 2025, Ideogram 3.0 offers groundbreaking text-to-image AI advancements for developers and creators. This iteration stands out with enhanced features, focusing on photorealistic rendering, superior text integration, and enhanced creative direction.
Key Advancements for Developers
Developers will appreciate Ideogram 3.0's improved API, facilitating seamless integration into diverse workflows. The model's enhanced visual fidelity is evident in its depiction of human figures and complex textures, perfect for applications requiring high-quality image generation. With the inclusion of diverse ethnic representation, developers can create more inclusive and realistic digital content. The model's remarkable typography capabilities allow for the generation of intricate textual layouts ideal for professional-grade outputs like logos and marketing materials. Integrating these features into web and UI design projects can significantly improve user experience and project execution speed.
Creative Opportunities for Designers
For creators, Ideogram 3.0 unlocks new artistic possibilities. It empowers users to apply style references from 4.3 billion presets, ensuring aesthetic consistency across projects. The ability to experiment with the random style feature and save preferred styles through Style Codes enhances creative workflow flexibility. This allows for efficient production of cohesive brand materials, compelling marketing visuals, and diverse digital content. The effortless synthesis of style control with advanced photorealism supports creators in crafting visually striking content that resonates with varied audiences.
Strategic Implementation
To maximize Ideogram 3.0's potential, users should leverage its batch generation capabilities for efficient content scaling and diversity in representations. By optimizing text prompts and utilizing style references, professionals can maintain aesthetic consistency, ensuring each project meets its unique stylistic and representational needs.
Mastering Ideogram 3.0: A Quick-Start Guide
Ideogram 3.0 delivers industry-leading photorealism, advanced typography, and seamless style control. Use this guide to craft precise prompts, optimize parameters for different workflows, and unlock the full potential of text-to-image generation.
1. Crafting Your Prompt
• Be specific: include subject, environment, mood, lighting, and focal details (“A modern workspace with wooden desk, soft afternoon light, potted succulents, and MacBook Pro”).
• Leverage positive and negative prompts: highlight what you want, then exclude unwanted elements (“No clutter, no people”).
• Use Magic Prompt (AUTO
/ON
) for complex scenes; switch OFF
when you need full manual control.
2. Core Parameters Overview
• Resolution (e.g. 1024x1024
): higher → more detail; lower → faster.
• Aspect Ratio (1x1
, 16x9
, etc.): frame your composition for social, widescreen, or print.
• Rendering Speed (TURBO
, DEFAULT
, QUALITY
): balance speed vs. fidelity.
• Seed: fix for reproducible results; omit for endless variations.
• Style Type (REALISTIC
, DESIGN
, GENERAL
): match your creative intent.
• Color Palette: choose predefined or custom hex values to enforce brand colors.
• Style Codes: save or apply codes from prior runs for consistent aesthetics.
3. Use-Case Parameter Recipes
-
Photorealistic Marketing & Advertising (Brand Assets)
- Resolution:
1536x640
or1536x576
- Aspect Ratio:
16x9
or4x3
- Rendering Speed:
QUALITY
- Magic Prompt:
ON
- Style Type:
REALISTIC
- Color Palette: Your brand’s hex values
- Negative Prompt: “No noise, no distortion”
- Resolution:
-
Rapid Concept Sketches & Prototyping
- Resolution:
832x1024
or896x1152
- Aspect Ratio:
3x4
or2x3
- Rendering Speed:
TURBO
- Magic Prompt:
AUTO
- Style Type:
GENERAL
- Seed: omit for varied outputs
- Resolution:
-
Typography & Logo Design
- Resolution:
1024x1024
- Aspect Ratio:
1x1
- Rendering Speed:
DEFAULT
- Magic Prompt:
OFF
- Style Type:
DESIGN
- Include explicit text in prompt and adjust negative prompt: “No background patterns”
- Resolution:
-
Cinematic Scene Generation
- Resolution:
1280x704
or1408x576
- Aspect Ratio:
16x9
or21x9
(via cropping) - Rendering Speed:
QUALITY
- Magic Prompt:
ON
- Style Type:
REALISTIC
- Use Style Codes for filmic LUTs
- Resolution:
4. Pro Tips
• Batch requests: generate 4–8 variants per batch to explore diversity.
• Lock a seed and tweak one parameter at a time for controlled A/B testing.
• Save and reapply Style Codes to maintain visual cohesion across campaigns.
By combining careful prompt construction with targeted parameter settings, Ideogram 3.0 becomes your powerhouse for high-quality, consistent, and efficient image creation.
Other Popular Models
sdxl-img2img
SDXL Img2Img is used for text-guided image-to-image translation. This model uses the weights from Stable Diffusion to generate new images from an input image using StableDiffusionImg2ImgPipeline from diffusers
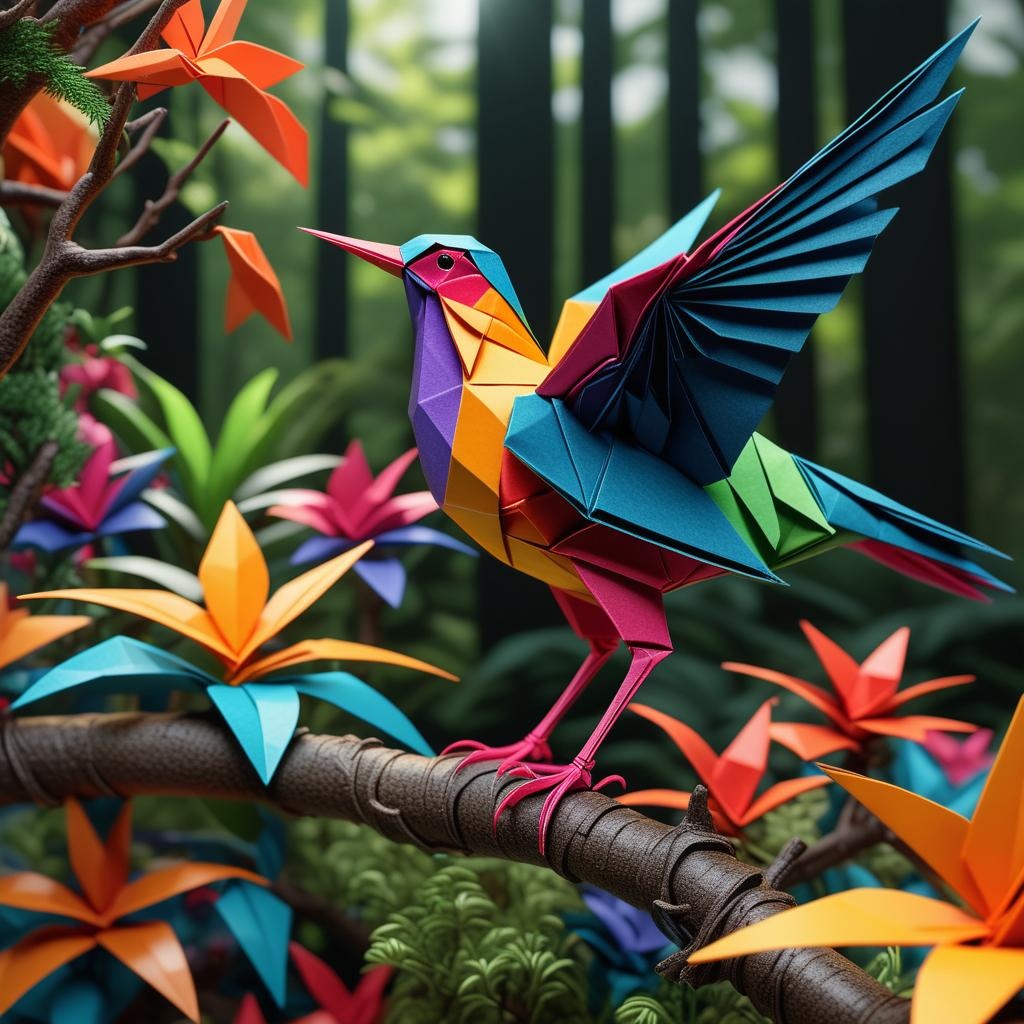
sdxl-controlnet
SDXL ControlNet gives unprecedented control over text-to-image generation. SDXL ControlNet models Introduces the concept of conditioning inputs, which provide additional information to guide the image generation process
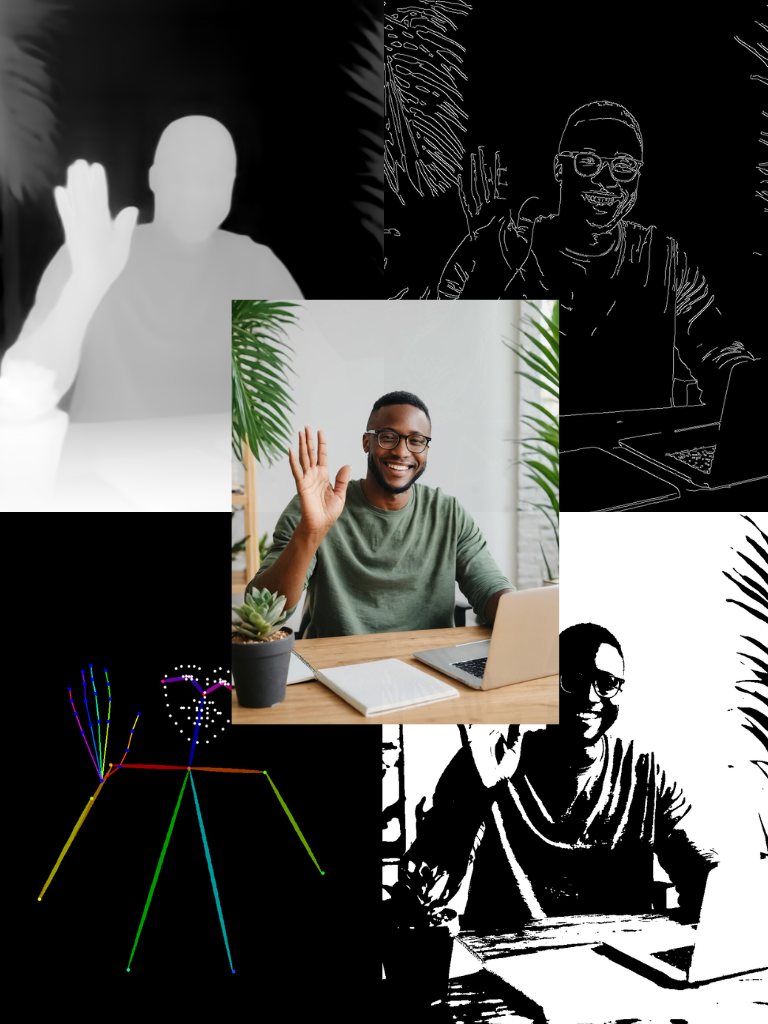
face-to-many
Turn a face into 3D, emoji, pixel art, video game, claymation or toy
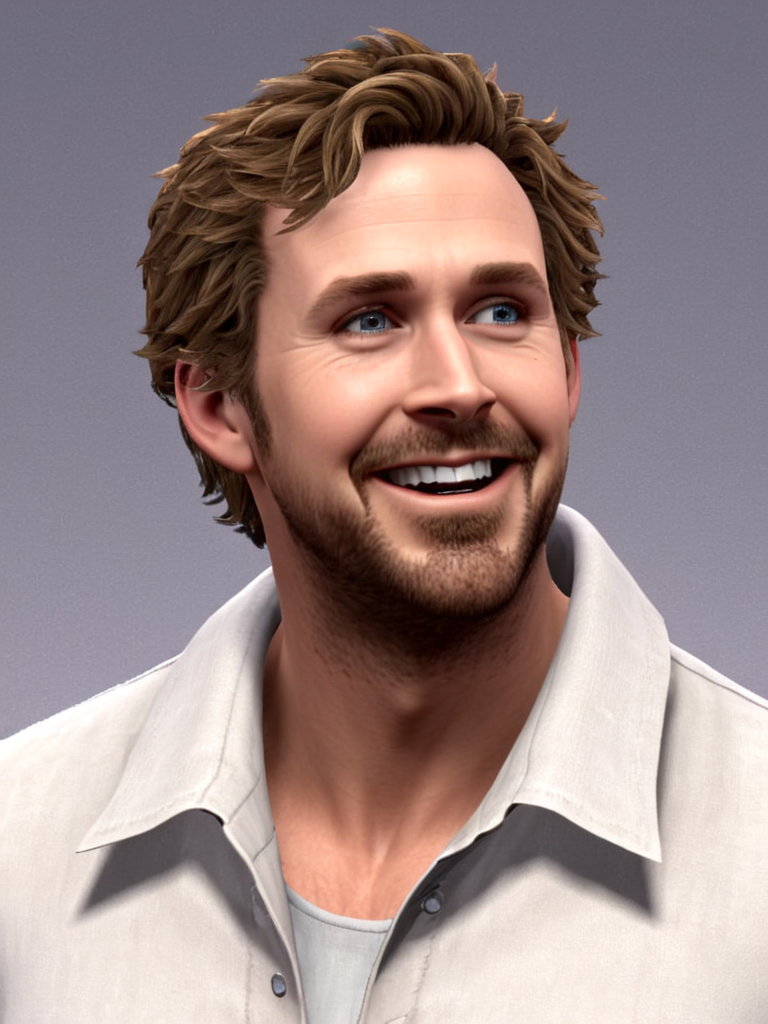
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
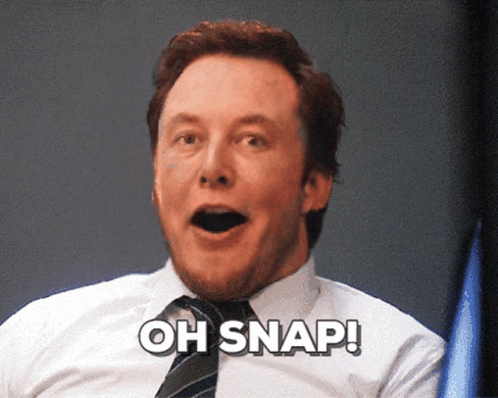