API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/nomos-upscaler"
# Request payload
data = {
"image": image_url_to_base64("https://segmind-resources.s3.amazonaws.com/input/557ae4e3-8057-4668-bf41-ff836d0f73b0-test_upscale_1234142.jpg"), # Or use image_file_to_base64("IMAGE_PATH")
"image_format": "png",
"image_quality": 95,
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
URL or base64 of input image
Format of the output image
Allowed values:
Quality of the output image (1-100)
min : 1,
max : 100
Return image as base64 string
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
About Nomos8k_span_otf_medium upscaler model
The Nomos8k_span_otf_medium AI model is a upscaler model developed that specializes in general image upscaling with a strong emphasis on realistic restoration, particularly for photographic content. It efficiently quadruples the resolution of your images, bringing out finer details while striving to maintain a natural look, avoiding artificial enhancements or excessive smoothing.
Built upon the relatively fast SPAN architecture, Nomos8k_span_otf_medium offers a significant speed advantage over some other upscalers like Waifu2x, making it a practical choice for processing numerous images quickly. Its training on the "nomos8k" dataset, utilizing an Online Transformation Function (OTF), has equipped it to handle common real-world degradations such as noise, JPEG and WebP compression artifacts, scaling blur, and even lens blur. This robust training means it's well-suited for enhancing photos you might encounter online or in your personal archives.
For effective use, remember that while designed for general photographic content, experimentation with other image types is possible. Consider the specific characteristics of your input image; for heavily compressed or very noisy images, other specialized models might yield slightly different results. However, for a balanced approach to realistic 4x upscaling with good speed, Nomos8k_span_otf_medium presents a compelling option. Its adoption within AI image generation workflows like Stable Diffusion's SUPIR and its compatibility with software backends like tensorrt further attest to its practical utility. Just remember, the "Nomos" in its name refers to its training data and is distinct from the "Nomos Sans" font family.
Other Popular Models
sdxl-img2img
SDXL Img2Img is used for text-guided image-to-image translation. This model uses the weights from Stable Diffusion to generate new images from an input image using StableDiffusionImg2ImgPipeline from diffusers
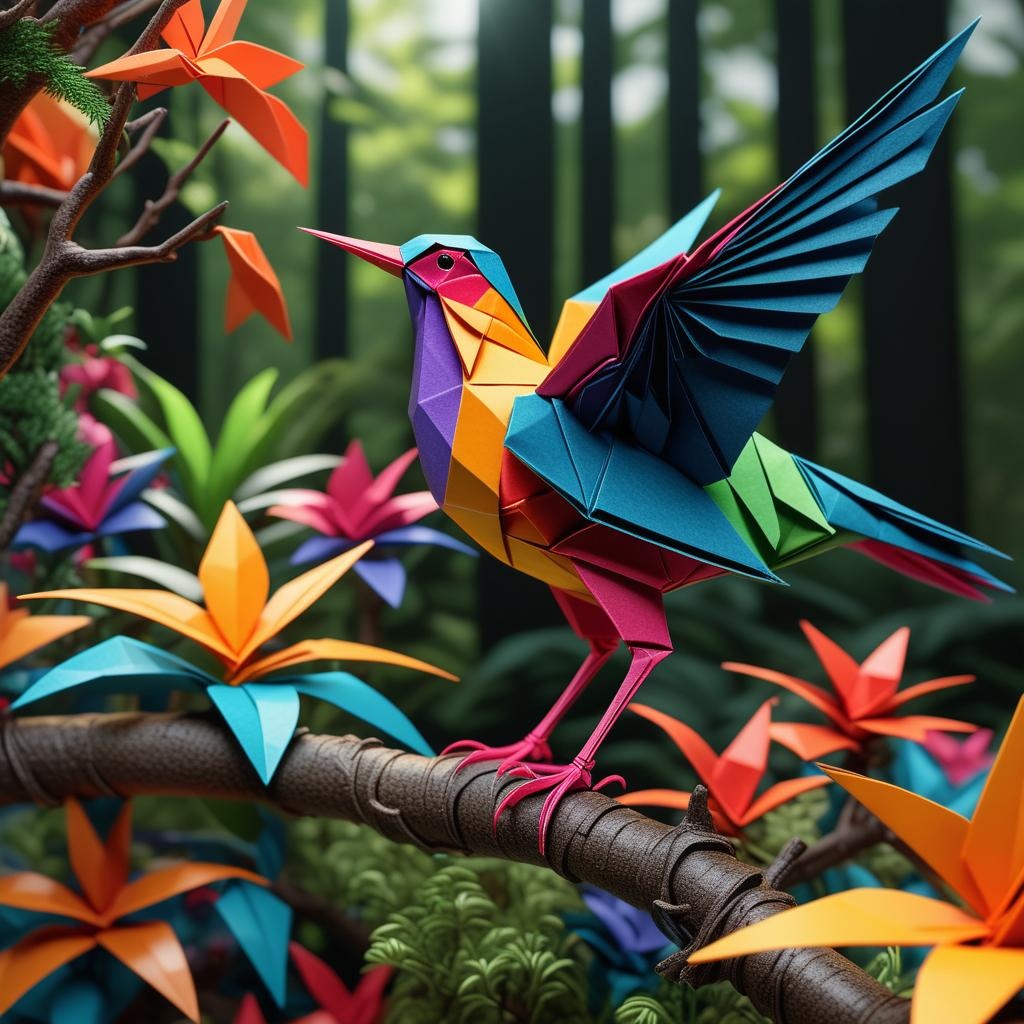
storydiffusion
Story Diffusion turns your written narratives into stunning image sequences.
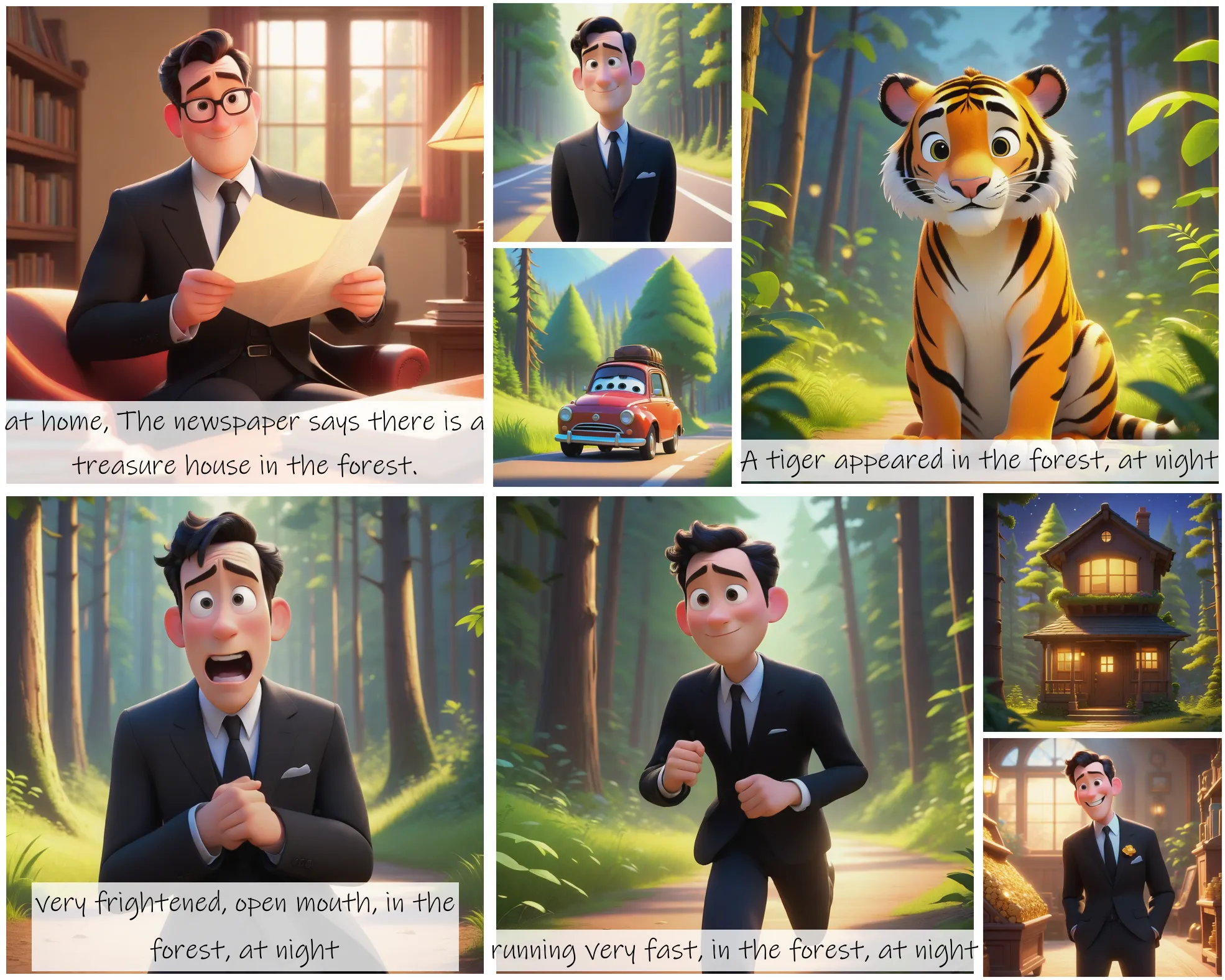
faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
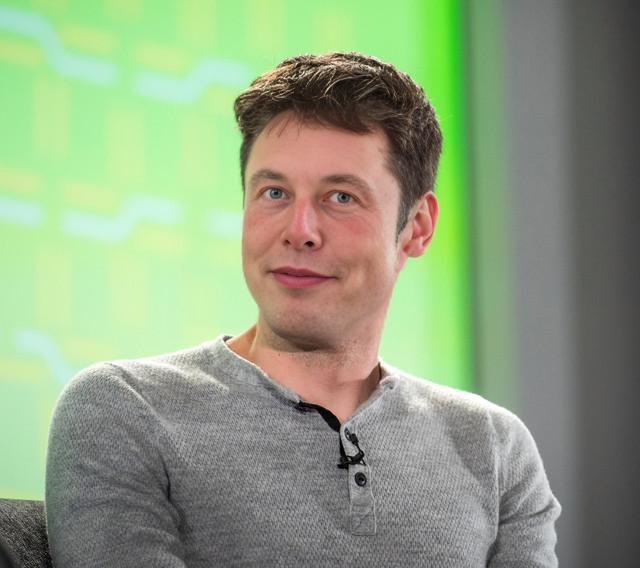
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
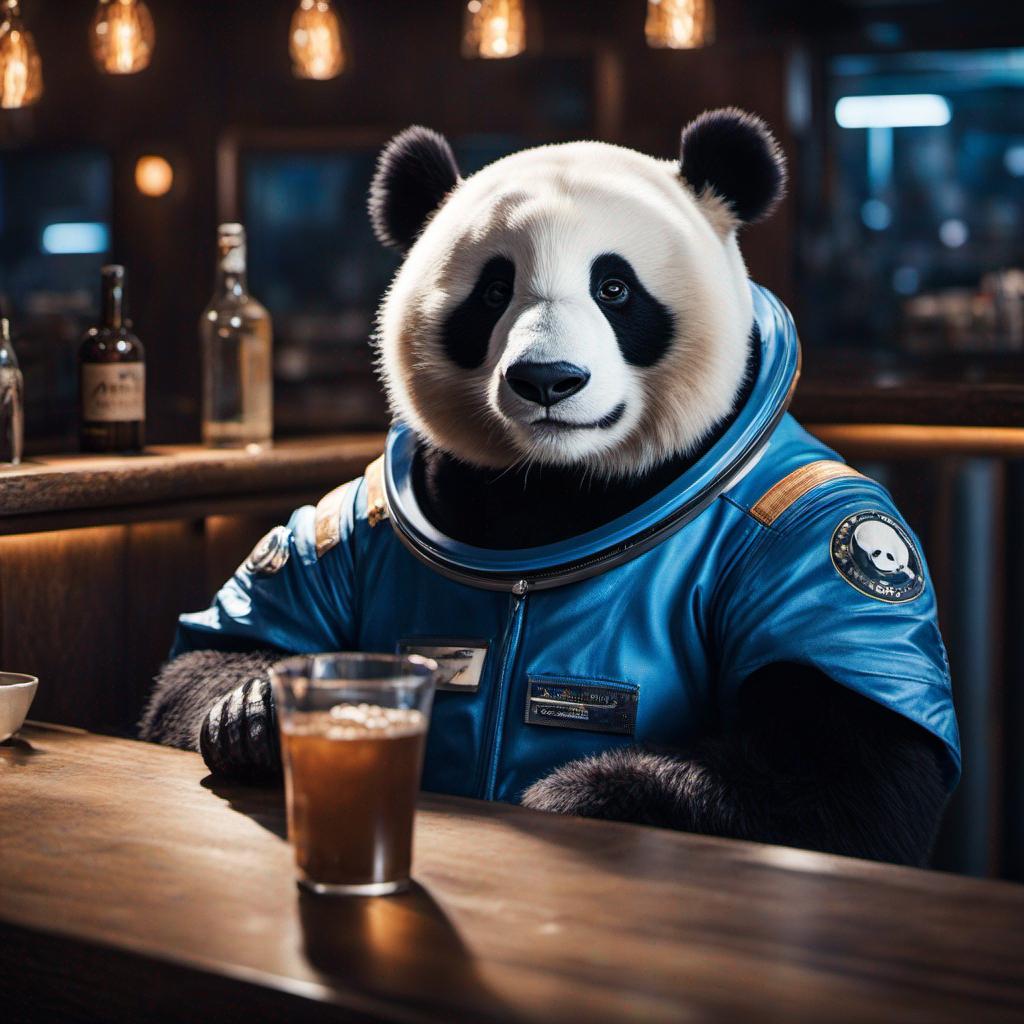